Featuring Jest, Karma, Chai, Vitest, and Cypress
Introduction to Unit Testing in Software Development
Let’s be honest—software development can feel like juggling flaming torches while riding a unicycle. Things break, deadlines loom, and bugs sneak in like uninvited guests at a party. This is where unit testing comes in to save the day (and your sanity).
What is unit testing?
Unit testing is about taking the smallest piece of your code—usually a function or module—and putting it under the microscope. You check if it behaves as expected, and if not, you have some debugging to do.
Importance of unit testing in software engineering
Think of it as a safety net. Unit tests make your code reliable, maintainable, and ready to grow with your ideas. They catch issues early, saving you from costly, time-consuming fixes down the line. Whether building the next big app or trying to survive your sprint planning, unit testing is a game-changer for all.
The Benefits of Writing Unit Tests: It’s Worth Your While
If you’re still unsure about writing unit tests, here’s what they bring to the table:
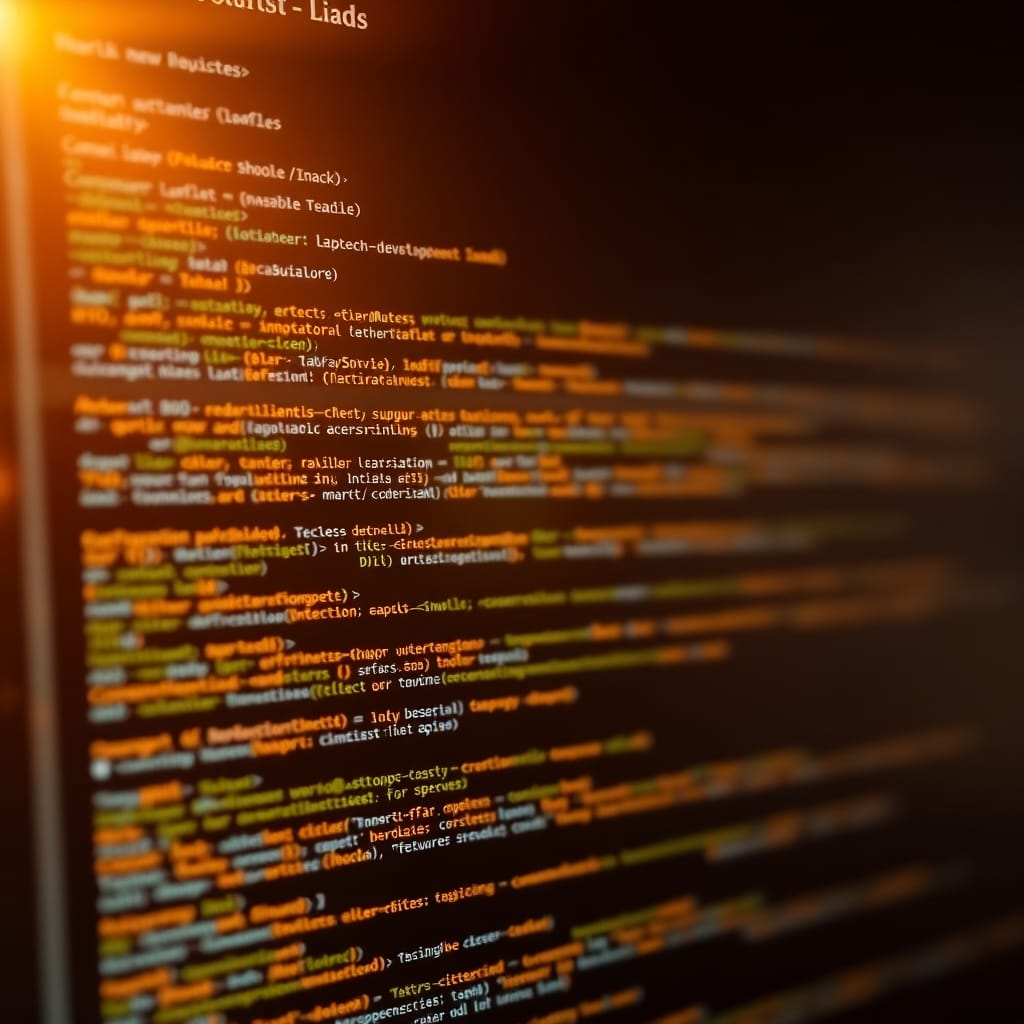
1. Improved code quality and maintainability
Writing unit tests forces you to write cleaner, more modular code. You’ll naturally break things down into smaller, testable chunks—making it easier to maintain and scale your application in the future.
2. Early bug detection and cost savings
Bugs caught early are cheaper to fix. Without tests, you might not realize something’s broken until it’s too late (and your boss is breathing down your neck). Unit tests help you spot issues before they snowball into disasters.
3. Streamlining refactoring and feature updates
Want to refactor that messy legacy code? No problem! Unit tests allow you to test new updates while saving you from breaking existing functionality—yet another reason why you should make them a part of your workflow. They allow you to experiment with confidence, knowing that you are covered against catastrophic failures.
4. Enhancing collaboration and onboarding
Adding new team members? No worries. Unit tests can speed up onboarding and foster better collaboration.
They serve as interactive documentation, showing new members exactly how different parts of the system should behave. You wouldn’t immediately associate this with unit tests, but once you experience it, you simply cannot imagine life without it.
5. Never Again See the Bug You Saw
Every bug can be squashed when accompanied with unit test. It will never appear again.
Popular JavaScript Unit Testing Frameworks
Developers are spoilt for choice when it comes to JavaScript testing frameworks. Here are some of the most common and popular options:
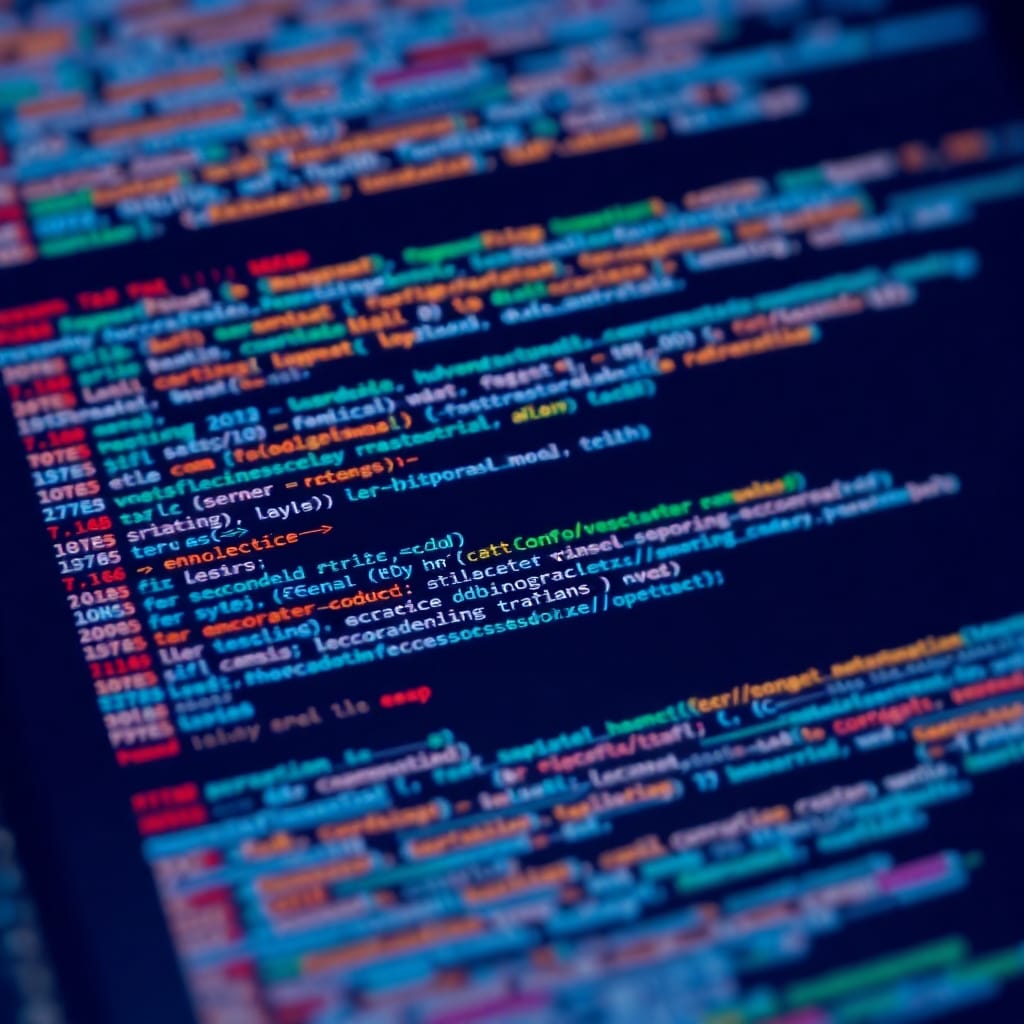
- Jest – The go-to choice for most developers. It offers a rich feature set and boasts built-in mocking capabilities.
- Karma is great for running tests in real browsers and front-end apps.
- Chai – Its powerful assertion library is highly compatible with other frameworks, making it popular for complex projects.
- Vitest – is a rising star known for its lightning-fast performance and great DX. If you are using Vuejs, you will get a lot done faster and effortlessly.
- Cypress – While primarily used for end-to-end testing, it also offers solid support for unit tests.
Each tool offers something unique, whether you’re looking for speed, ease of use, or compatibility with your existing stack.
Writing Unit Tests with Jest
If JavaScript testing had a popularity contest, Jest would be the cool kid. It’s fast, feature-rich, and has built-in utilities that make testing a breeze.
Why Jest is a popular choice
Jest has a zero-config approach, so you can start testing in minutes. Plus, it offers a few more features for the savvy coder—powerful mocking, snapshot testing, and an intuitive API—perfect for both beginners and seasoned developers.
Setting up Jest for your project
Getting started with Jest is as easy as:
npm install –save-dev Jest
Then, add a test script in your package.json:
"scripts": {
"test": "jest"
}
Run your tests with the following:
npm test
Writing and running basic tests
Here’s what a simple test might look like in Jest:
const sum = (a, b) => a + b;
test(‘adds 2 + 3 to equal 5’, () => {
expect(sum(2, 3)).toBe(5);
});
Boom! You’ve written your first unit test.
Using Chai for JavaScript Unit Testing
Chai is your new best friend if you love expressive, human-readable tests.
Introduction to Chai Assertion Library
Chai provides a range of assertion styles—should, expect, and assert—allowing you to write tests that feel natural and intuitive.
Combining Chai with other testing frameworks
Chai plays nicely with frameworks like Mocha and Cypress, making it a versatile choice for your testing stack.
Here’s a quick example using Chai with Mocha:
const chai = require(‘chai’);
const expect = chai.expect;
describe(‘Array’, function() {
it(‘should start empty’, function() {
const arr = [];
expect(arr).to.be.an(‘array’).that.is.empty;
});
});
Readable, right?
Best Practices for Effective Unit Testing: Getting Started on the Right Foot
Writing tests is great, but writing good tests is what you want to do. See below for some best practices to keep your unit tests effective and maintainable:
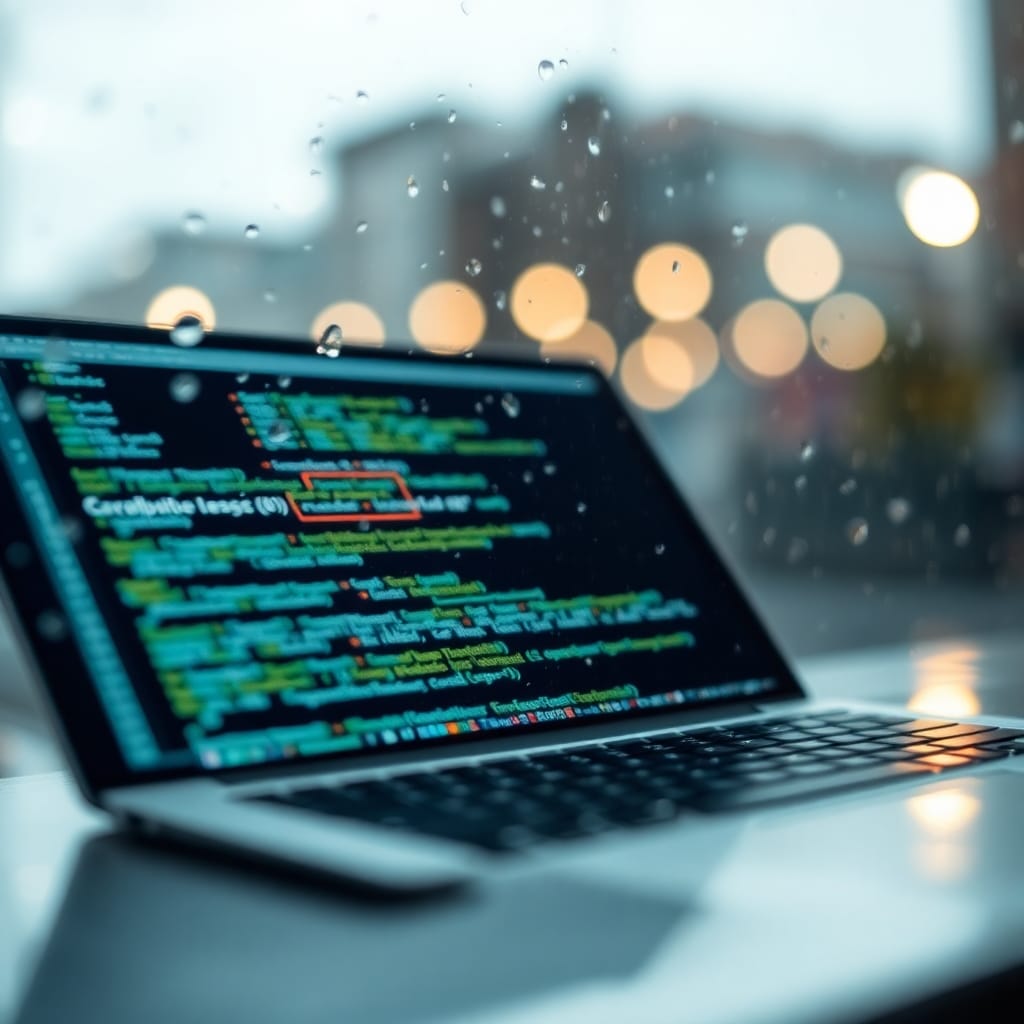
1. Embrace Test-Driven Development (TDD)
TDD forces you to consider requirements before writing code. Start by writing a failing test, make it pass, and then refactor. This disciplined approach really pays off as you go along.
2. Structure your tests for readability and maintainability
Use descriptive test names, organize tests logically, and avoid duplication. In the future, you (and your teammates) will thank you.
3. Avoid common pitfalls
Don’t over-test trivial things. Focus on what’s essential and core to your project. Keep tests independent of each other. Always mock external dependencies and avoid relying on global states.
Conclusion: Unlock Efficiency In Your Development Process with Unit Tests
By now, you should see how Unit testing is more than just a chore you are supposed to do. It is more like an investment in the long-term health of your project. Once you adopt a test-first mindset, your applications will become robust, easily maintainable, and scalable without effort. This will save you time, money, and effort down the road.
Just a few things your developers need to keep in mind:
1. Require developers to write tests that are designed to fail when necessary.
2. Avoid focusing on test coverage; it is merely a metric.
3. A unit test should accompany every bug fix.
4. Create a wrapper that makes writing unit tests easier for developers.
5. The easier it is to write unit tests, the more and better unit tests developers will produce.
Unit testing has an upfront cost as it increases development time but in the longer run, it can significantly save costs and make for a mature product that is stable and highly functional. Investors also love code that has good coverage.
So, what are you waiting for? Grab Jest, Chai, or any of the awesome tools mentioned above, and start writing tests that make your code bulletproof. Your future self will thank you.